Hiện nay ReacJS được xem là một trong những Front-end library mạnh mẽ và phổ biến nhất trên thế giới, bên cạnh là Angular và VueJS.
Cũng như VueJS và React, Svelte cũng là một component framework, tức là hướng tới việc build user interface từ các component.
1. SvelteJS là gì, Vì sao svelte có thể trở thành kẻ ngáng đường thách thức ReactJS
Trong khi React hay Vue sử dụng kĩ thuật DOM diffing, cho phép developer viết declarative state-driven code rồi buộc trình duyệt phải làm nhiều việc hơn để convert declarative structures ở trên thành các DOM operation.
Thay vào đó, SvelteJS chuyển toàn bộ công việc đó vào trong 1 complie step, convert các component của bạn thành highly efficient imperative code mà có thể cập nhật được DOM. Điều này giúp chúng ta có thể viết được những ứng dụng với excellent performance.
Trích dẫn từ trang chủ Svelte:
Svelte is a radical new approach to building user interfaces. Whereas traditional frameworks like React and Vue do the bulk of their work in the browser, Svelte shifts that work into a compile step that happens when you build your app.
Instead of using techniques like virtual DOM diffing, Svelte writes code that surgically updates the DOM when the state of your app changes.
Có thể coi Svelte như là 1 compiler hơn là 1 library. Svelte chạy ở build time và convert component thành plain JavaScript efficient code
Trong phần tiếp theo của bài viết này, chúng ta sẽ đi lần lượt so sánh React và Svelte ở một số điểm như sau
2. Khởi tạo project ReactJS và SvelteJS
Trước tiên ta sẽ xem cách khởi tạo project khi dùng React và Svelte. Hiện tại, cách đơn giản nhất để khởi tạo một dự án React và Svelte là sử dụng tool Create React App và degit
Trên terminal ta chạy lệnh sau
$ npm install -g create-react-app
$ npm install -g degit
Sau khi cài đặt tool xong, chúng ta tiến hành khởi tạo project.
Đối với React:
Ta chạy lệnh sau:
$ create-react-app myreactapp
Sau khi project được tạo, chúng ta chạy lệnh sau để khởi động project
$ cd myreactapp && npm start
App sẽ có trên http://localhost:3000
bạn thấy hình sau
Đối với Svelte
Để khởi tạo project Svelte, ta chạy lệnh sau:
$ npx degit sveltejs/template sveltenewsapp
Tiếp theo, dùng lệnh sau để cài đặt dependencies và khởi động
$ cd sveltenewsapp
$ npm install
$ npm run dev
App sẽ có trên http://localhost:5000
như sau:
Có thể thấy rằng, cả hai đều có thể dễ dàng khởi tạo project bằng các tool cài đặt sẵn.
3. Component Oriented
Tiếp theo, chúng ta sẽ tìm hiểu xem cách tạo component từ React và Svelte
Trong Frontend, component được hiểu như là những đoạn code có thể tái sử dụng được và là những phần tạo nên user interface. Cụ thể hơn nó bao gồm các function hoặc class Javascript để xử lý logic, HTML để render và CSS để styling. Component chính là building block
trong ứng dụng React lẫn Svelte.
Trong React, để tạo 1 component chúng ta có thể:
- Khai báo 1 class mà extend
React.Component
và có thể sử dụng các lifecyle-events và state
import React from 'react'; class MyComponent extends React.Component { constructor(props) { super(props); this.state = { //state here }; } render() { return ( <div> // Render your component </div> ) } }
- Hoặc cũng có thể sử dụng hàm và các hook để tạo component và quản lý state
import React, { useState} from 'react'; function MyComponent(props){ const [state, setState] = useState('state'); return ( <div> // Render your component </div> ) }
Đối với Svelte
Để tạo component ta sẽ viết những file .svelte
bao gồm tag <script>
, <style>
và markup
<script> export let name; </script> <main> <h1>Hello {name}!</h1> <p>Visit the <a href="https://svelte.dev/tutorial">Svelte tutorial</a> to learn how to build Svelte apps.</p> </main> <style> main { text-align: center; padding: 1em; max-width: 240px; margin: 0 auto; } h1 { color: #ff3e00; text-transform: uppercase; font-size: 4em; font-weight: 100; } @media (min-width: 640px) { main { max-width: none; } } </style>
Như vậy ta đã tạo được 1 component App với Svelte
4. Fetch và hiển thị dữ liệu
Trong phần tiếp theo chúng ta sẽ thấy các fetch và hiển thị dữ liệu của React và Svelte.
Đối với React
Ta tạo 1 file src/App.js
như sau:
import React from 'react'; import './App.css'; function App() { const apiKEY = "<YOUR-API-KEY>"; const dataUrl = `https://newsapi.org/v2/everything?q=javascript&sortBy=publishedAt&apiKey=${apiKEY}`; const [items, setItems] = React.useState([]); const fetchData = async () => { const response = await fetch(dataUrl); const data = await response.json(); console.log(data); setItems(data["articles"]); }; React.useEffect(() => { fetchData(); }, []); return ( <> <h1> Daily News </h1> <div className="container"> { items.map(item => { return ( <div className="card"> <img src= { item.urlToImage } /> <div className="card-body"> <h3>{item.title}</h3> <p> {item.description} </p> <a href= { item.url}>Read</a> </div> </div> ); }) } </div> </> ); } export default App;
Tạo tiếp file src/App.css
để style cho component App
h1 { color: purple; font-family: 'kalam'; } .container { display: grid; grid-template-columns: repeat(auto-fill, minmax(305px, 1fr)); grid-gap: 15px; } .container > .card img { max-width: 100%; }
Tiếp theo ta sẽ làm tương tự với Svelte
Tạo 1 file src/App.svelte
như sau:
<script> import { onMount } from "svelte"; const apiKEY = "<YOUR-API-KEY>"; const dataUrl = `https://newsapi.org/v2/everything?q=javascript&sortBy=publishedAt&apiKey=${apiKEY}`; let items = []; const fetchData = async () => { const response = await fetch(dataUrl); const data = await response.json(); console.log(data); items = data["articles"]; }; onMount(fetchData()); </script>
Tiếp đến ta thêm code HTML để render dữ liệu bên dưới tag script
<script> import { onMount } from "svelte"; const apiKEY = "<YOUR-API-KEY>"; const dataUrl = `https://newsapi.org/v2/everything?q=javascript&sortBy=publishedAt&apiKey=${apiKEY}`; let items = []; const fetchData = async () => { const response = await fetch(dataUrl); const data = await response.json(); console.log(data); items = data["articles"]; }; onMount(fetchData()); </script> <h1> Daily News </h1> <div class="container"> {#each items as item} <div class="card"> <img src="{item.urlToImage}"> <div class="card-body"> <h3>{item.title}</h3> <p> {item.description} </p> <a href="{item.url}">Read</a> </div> </div> {/each} </div>
Và cuối cùng ta thêm tag style tương tự để styling
<script> import { onMount } from "svelte"; const apiKEY = "<YOUR-API-KEY>"; const dataUrl = `https://newsapi.org/v2/everything?q=javascript&sortBy=publishedAt&apiKey=${apiKEY}`; let items = []; const fetchData = async () => { const response = await fetch(dataUrl); const data = await response.json(); console.log(data); items = data["articles"]; }; onMount(fetchData()); </script> <h1> Daily News </h1> <div class="container"> {#each items as item} <div class="card"> <img src="{item.urlToImage}"> <div class="card-body"> <h3>{item.title}</h3> <p> {item.description} </p> <a href="{item.url}">Read</a> </div> </div> {/each} </div> <style> h1 { color: purple; font-family: 'kalam'; } .container { display: grid; grid-template-columns: repeat(auto-fill, minmax(305px, 1fr)); grid-gap: 15px; } .container > .card img { max-width: 100%; } </style>
Nhận xét:
- Như ta có thể thấy, cả 2 app đều có URL và API key để gọi API lấy dữ liệu về
- Đối với React, ta sử dụng 1 biến là
items
bằng cách dùng hookuseState
, trong khi Svelte, chỉ cần dùng từ khóalet
để khai báo choitems
. - Khi state thay đổi, cả 2 đều re-render, tuy nhiên nếu React cần có 1 method để set lại giá trị của state thì Svelte không cần. Mặc định chính bản thân biến trong Svelte là reactive state.
- Điều này có thể thấy rõ khi fetch xong data từ API về. React cần phải có method
setItems
tạo từ hook để cập nhật lại giá trịitems
thì Svelte chỉ cần dùng phép gán - Để xử lý side effect khi gọi hàm
fetchData()
khi component được tạo, React sử dụng hook useEffect. Tương tự Svelte cũng dùng hàmonMount
để gọi hàm khi component mounted.
Kết
Nói tóm lại, dù cả ReactJS và SvelteJS đều dùng các concept của component như: state, life-cycle method cũng như props, nhưng mỗi bên lại dùng theo một cách khác nhau: SvelteJS là 1 build-time compiler trong khi ReactJS lại là run-time library và cả 2 đều cung cấp những công cụ để nhanh chóng tạo xây dựng User Interface cho project.
Reference:
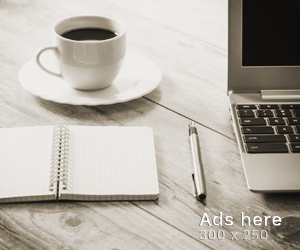